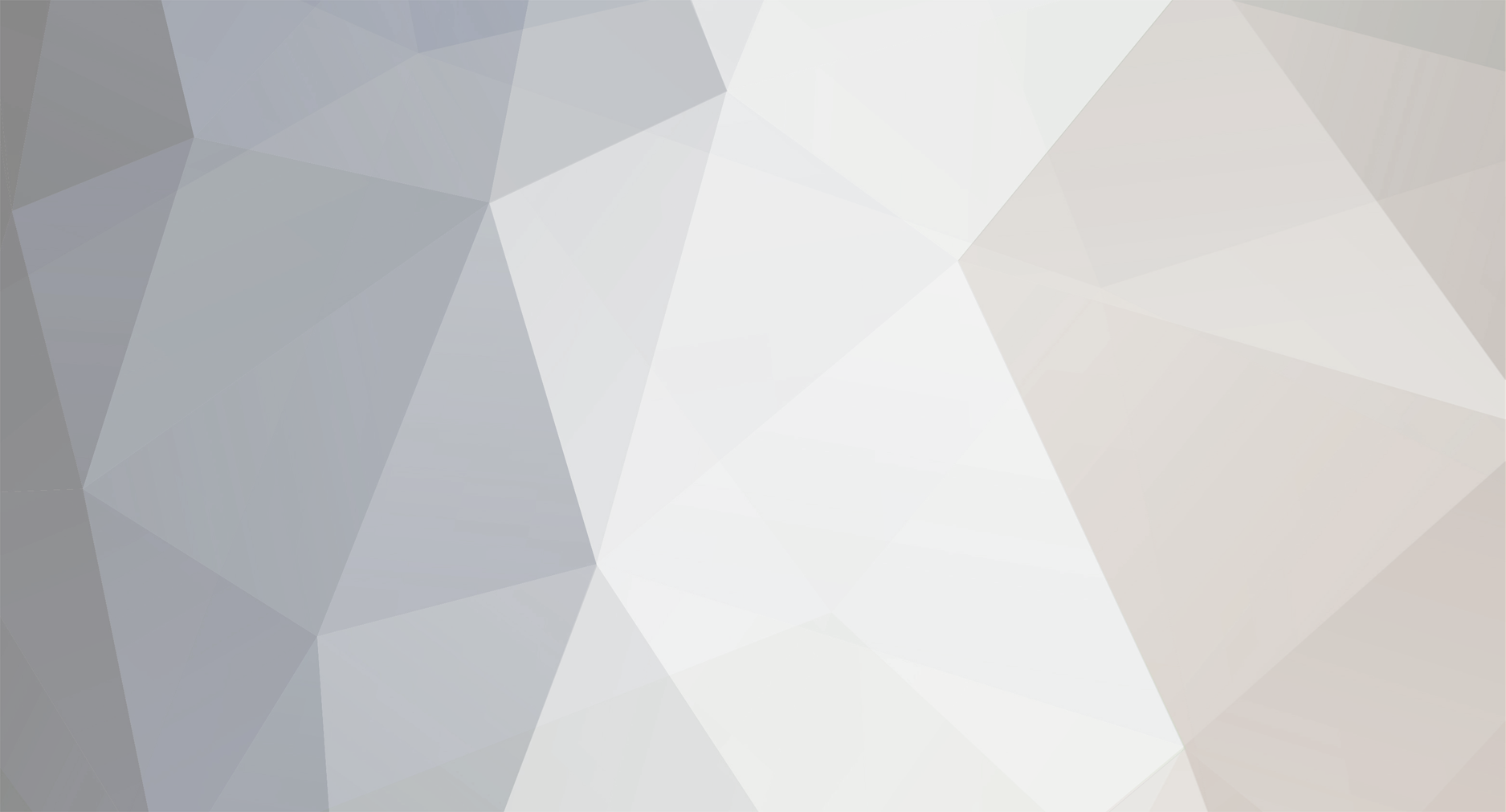
grimfang4
Members-
Posts
52 -
Joined
-
Last visited
Content Type
Forums
Store
Gallery
Downloads
Everything posted by grimfang4
-
Flipping seems to work okay over here, except that I haven't added lucid's angle suggestion to fix the rotation when one dimension is scaled negatively (this problem only appears because we're not using matrix math). EDIT: It's added now, though uneven scaling (squishing and stretching) isn't perfect. I take no offense, but do ask others to evaluate code realistically. If a performance issue is really observed, then that's when something needs to be done about it. Please let me know right away if that's the case. A library written in C is very useful for having a common ABI between languages, but it's really not going to have any sort of significant performance benefit. The time needed to develop and maintain it would be higher, as well. If there's anything in particular that you think is too heavy in SCMLpp, please tell me and I can either improve that or make it more modular so it can be replaced easily. I know the code doesn't look particularly simple right now, but precise feedback always helps.
-
Maybe it is the angle calculation? The one you posted looks suspicious because they shouldn't be deltas. You should be interpolating (tweening/lerping) between them. Here's the relevant code (edited slightly) from SCMLpp.cpp line 2628: if(prev_spin != 0) { if(prev_spin > 0 && prev_angle > next_angle) prev_angle = lerp(prev_angle, next_angle + 360, t); else if(prev_spin < 0 && prev_angle < next_angle) prev_angle = lerp(prev_angle, next_angle - 360, t); else prev_angle = lerp(prev_angle, next_angle, t); } 't' is the interpolation ratio (a free parameter): t = (current_time_in_animation - prev_time)/float(next_time - prev_time); And lerp() is defined like so (SCMLpp.cpp line 2452): inline float lerp(float a, float b, float t) { return a + (b-a)*t; }
-
As a quick start, here's a video of a couple of those errors: http://youtu.be/rl6QEidFRFM Shown there are inverted y and inverted angle errors.
-
What we mean is a base scaling of the entire sprite, including bone/object positions and everything. You can see it in my implementation as the base_transform (line 2483 in SCMLpp.cpp). The info used to create this transform is provided by the program and is used to position, rotate, and scale the entire sprite dynamically so it fits in a game.
-
I've been considering which features should be implemented in SCMLpp (the C++ API). Let's put our heads together and maybe we can get a good feel for what features any engine API would benefit from. Here are my ideas. I'll leave out the ones which are in the SCML spec, but not yet implemented in the plugins. * Bone drawing * Animation blending * Runtime bone control * Runtime inverse kinematics * Object transform accessor Bone drawing: Mostly useful for debugging, but also for effects. For debugging purposes, drawing just a line for bones and a dot for joints works fine. For effects, there could be a way to ask the library for a list of the bone positions so it can use them however it wants. Animation blending: This is an important feature. It's not yet supported by Spriter (or SCML). What needs to happen is to identify the same objects between animations. Then one animation can lead into another smoothly. There are control issues that may need to be resolved, since it's common for automatic transitions to look strange (e.g. feet lifting off the ground during the transition). Runtime bone control: Simple control of bone parameters. Position, angle, etc. This can probably be integrated into the tweened animation process by creating temporary keyframes. Runtime inverse kinematics: Controlling a target instead of the individual bones. There are probably several parameters that might need to be controlled here, like constraints and joint directions. I've never messed with IK before... This could also use the temporary keyframes that the runtime bone control use. Object transform accessor: Get the absolute position, angle, etc. of a given object. Do you have any other ideas or input on these?
-
I think character maps are a very important feature that would get used right away. Being able to use the same animation for multiple different characters or swapping body parts seems more important than atlases (an optimization), at least. I could make an interface for those features in the C++ API directly, but I'd rather make it compatible with the planned SCML spec. A well-designed generic API is one that lets you do that without code changes to the actual library. If there are clear, flexible, and comprehensive interfaces to the data, you do not need to break the API to make it fit your engine. I just need community input to map out those interfaces fully.
-
Yes, scaling the x-dimension by -1 is the usual way.
-
@shabby A C++ API is available already and will eventually include many features as you describe in (6). It loads and renders animations now. In the future (sooner if you can provide direct feedback), it will support various ways to manipulate animations. viewtopic.php?f=3&t=1133
-
You should take a look at my C++ library. It should be pretty easy to translate to Java or take whatever logic you need. viewtopic.php?f=3&t=1133
-
Those images look similar to my early results. The second one in particular I fixed by offsetting the pivot points by half the image dimensions.
-
There are three sample SCML projects in my repository that you're welcome to, if it helps. One is the monster, another is the hero, and the other is a knight with bones. viewtopic.php?f=3&t=1133 As for NPOT textures, this should be handled by your software in some form. With straight OpenGL, I load images into POT textures and save the width and height for adjusting the texture coords when accessing the texture.
-
[request] Simple Example for Coders
grimfang4 replied to mrwonko's topic in Spriter Animated Art Packs
I agree that it'd be great to have a more realistic test case. I was just pointing out that there won't be many features in such a sample yet and that you can take whatever I've collected so far in case it helps. -
[request] Simple Example for Coders
grimfang4 replied to mrwonko's topic in Spriter Animated Art Packs
I think there aren't a lot of SCML features implemented in Spriter yet. The important ones are there, like tweening and bones, but character maps, atlases, temp bones and objects, and the rest are not ready. My implementation, SCMLpp, is up-to-date with Spriter A4.1 and includes several C++ renderers. The SCMLpp.cpp source file currently contains all of the SCML loading (SCML::Data) and all of the animation logic (SCML::Entity). There are three sample SCML files, one of which has bones. It's worth taking a look at and I'm wide open to collaboration. viewtopic.php?f=3&t=1133 -
Yep, I figured out what the problem was. I was assuming that the mainline object ids could be used to refer to the same object in different keys, but Spriter actually follows the timeline for each object to do tweening. I fixed SCMLpp to use the timeline for that purpose. Problem solved. The Cocos2d-x renderer technically works, but I'll make it official as soon as it works as well as the others. There are still some kinks to smooth out.
-
I've just added some bone transform caching. Now each bone transform is calculated only once per draw.
-
Also, I just finished adding an SFML 2.0 renderer and test code. That makes three completed renderers so far: SDL_gpu, SPriG (no GPU), and SFML.
-
Thanks Obg1. It'll be great to have Cocos2d-x support. If you write the renderer code, you can email it to me or if I have your Google account email address, I can add you to the repository project members. The renderer interface draw_internal() is meant to rotate about and draw at the center of the image. This is the simplest renderer-agnostic choice. All the hard work is done elsewhere so that this function can be just a dumb drawing wrapper. I've fixed up the tweening so it doesn't crash on Hero.SCML (now included in the repo). However, it looks like there's a spin direction issue still...
-
How do bones effect the calculation of an object's position
grimfang4 replied to PatS's topic in Help and Tutorials
I think lucid is working on some more documentation that will illuminate further. In the meantime, I put together a SPriG (software SDL) renderer for SCMLpp. You can find it in the SCMLpp repository. SPriG should run on nearly anything. Do you have any specific questions? Is it a procedure for the parent transformations that you're after? For the transforms, SCMLpp r41 does that in SCML::Entity::draw() on line 2471 of SCMLpp.cpp and SCML::Entity::draw_tweened_object() on line 2600 of the same file. It's not totally cleaned up yet, but it demonstrates a naive implementation which works. Spriter/SCML does not use matrices for these transforms, so you do need to go through specific steps to get there. The basic idea (as I see it) is to take the child position, scale it by the accumulated scale_x and scale_y, then rotate it about the origin by the accumulated angle, then translate it by the parent position. After that, you combine the transforms by adding the angles, multiplying the scales, and set the parent/accumulated position to the new child position. -
For those who might want to create a new renderer for SCMLpp, I've just factored out all of the SCML complexity so the renderer implementation is incredibly simple. Take a look at SCML_SDL_gpu.h and SCML_SDL_gpu.cpp. The overall interface is still evolving, but the renderer-specific interface is settling down. If you want to contribute a renderer to the project and source repository, let me know!
-
You're welcome. It'll be very useful personally, once my projects are ready for it. The knight sprite is indeed crayon. My daughter drew it (and others) for my little game, Button Battle (http://www.dinomage.com/?s=button+battle). I didn't use Spriter for Button Battle, so it has very simple movements. To retain the authentic messy crayon style, I used the GIMP to cut a white layer to the shape of the sprite and the Color to Alpha tool (in the Colors menu) to overlay the drawing on top of the cutout.
-
Thanks to Edgar and Spriter A4! SCMLpp now draws entities that have bone hierarchies. A sample is included in the source repository. The sample and code might also be helpful to developers who are implementing bone animation in other plugins (more so when it gets cleaned up). I'll put together some extra documentation for such an implementation.
-
Alt+click is for creating bones. I think there's a tutorial video coming real soon.
-
It seems that in the latest Spriter alpha (a3), file paths below the project directory are specified as absolute (e.g. "/home/me/image.png" or "c:\Users\me\image.png"). In the interest of portable SCML files, I think all paths in SCML should be relative to the project directory. Perhaps in the cases where this is not possible (because separate Windows drives have distinct prefixes), the resources could be copied to the project directory or else the user is notified of the problem via popup and asked to copy the files to the same drive.
-
Thanks, Mike! I just fixed the "drawing mostly right" issue (you can see it in the previous image where some joints don't seem to line up - notably the forward knee). Tweening of the basic positioning parameters is implemented now too, so the animation is nice and smooth. So, except for comprehensive use cases, it's ready to be put into a game. I'm just awaiting an example that uses bones so I can finish that part. Then there are all the details left, like atlases, character maps, metadata and such. Here's to Spriter!
-